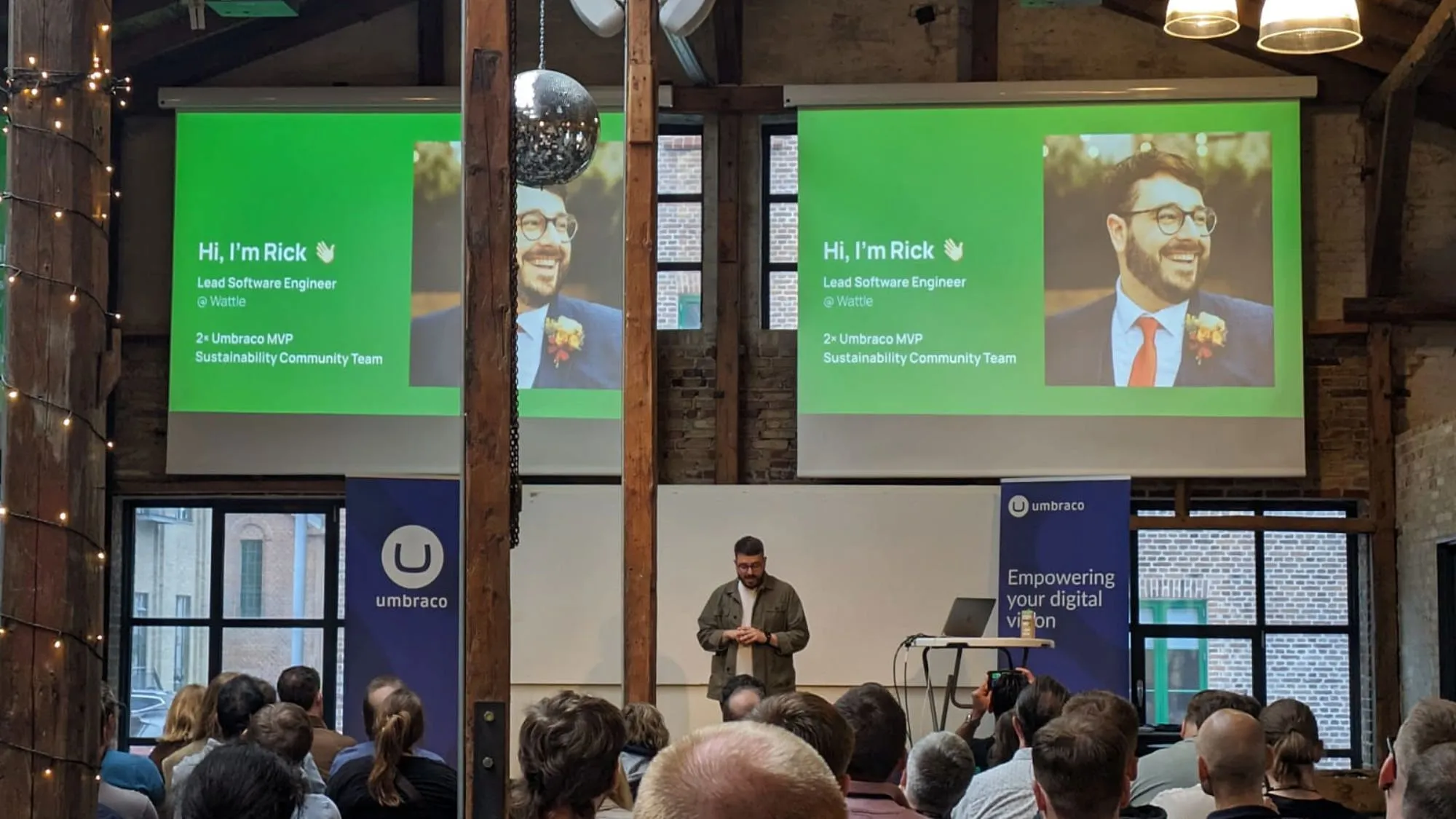
Hey there! 👋
I'm Rick, and I'm a full stack lead software engineer from the UK.
I'm currently working as Lead Software Engineer for Wattle where I get to build websites and software. This means I'm working across tech stacks—C# .NET, SQL and Umbraco as well as HTML, CSS/Sass, JS/ES6+ and Node.
I'm a 3× Umbraco MVP, Umbraco Certified Master and part of the Umbraco Community Sustainability Team.